DNS (Domain Name System) is an essential component of the internet, responsible for translating domain names into IP addresses to enable seamless communication between devices. However, its critical role also makes it a frequent target for malicious activity. Analyzing DNS logs can help detect security threats such as DNS tunneling — a covert method used to exfiltrate data by abusing DNS queries.
This article aims to guide readers through implementing a Python-based solution for DNS query analysis. The focus is on understanding the structure of DNS logs, detecting potential threats, and visualizing key patterns to gain actionable insights.
Purpose
DNS query analysis is pivotal for network security. By scrutinizing DNS logs, organizations can identify anomalies such as:
- Excessively long domain names, often a hallmark of DNS tunneling.
- Unusually high query frequencies, indicative of suspicious activity.
- Patterns that deviate from normal behavior, such as repeated queries to specific domains.
Python, with its robust libraries for data manipulation and visualization, is a powerful tool for this purpose.
Problem Statement
DNS tunneling poses a significant challenge as it allows attackers to bypass traditional security measures by hiding malicious traffic within legitimate DNS queries. Left undetected, this can lead to data breaches, malware proliferation, and other security risks.
Scope
In this article, readers will learn:
- How to simulate and generate a realistic DNS log dataset.
- Techniques to analyze DNS logs using Python libraries like pandas, matplotlib, and seaborn.
- Visualization methods to highlight anomalies and potential threats.
- Steps to interpret results and derive meaningful insights.
Prerequisites
To follow along, readers should have:
- A basic understanding of Python programming.
- Familiarity with DNS concepts and their role in networking.
- A Python environment set up, such as Jupyter Notebook or an IDE like PyCharm.
By the end of this guide, readers will have a functional Python-based framework for DNS query analysis, equipped to identify and respond to potential security threats effectively.
Understanding DNS and Security Threats
DNS (Domain Name System) is the backbone of the internet, converting human-readable domain names like example.com into machine-readable IP addresses. While it plays a critical role in facilitating online communication, DNS can also be exploited for malicious purposes.
DNS Overview
DNS operates as a hierarchical system, where domain names are resolved through iterative queries across multiple DNS servers. For example, when a user enters example.com, the query passes through:
- Recursive Resolver: Receives the query and acts as an intermediary.
- Root Server: Provides directions to the relevant Top-Level Domain (TLD) server (e.g., .com).
- TLD Server: Directs the query to the authoritative DNS server for the domain.
- Authoritative Server: Returns the IP address for the domain.
This process is efficient, but its openness also introduces vulnerabilities.
Common DNS Threats
DNS is often abused due to its ubiquitous nature and permissive design. Common threats include:
- DNS Tunneling
- Data is encoded into DNS queries and responses, enabling attackers to bypass firewalls or exfiltrate sensitive information covertly.
- Example: A long and suspicious domain like data.exfiltration.malicious.xyz.
- DNS Amplification Attacks
- Attackers exploit DNS servers to amplify their attack traffic in Distributed Denial-of-Service (DDoS) attacks.
- DNS Cache Poisoning
- Manipulating the DNS cache to redirect users to malicious sites.
- Phishing and Malware Distribution
- Malicious domains are created to deceive users or host malware.
Key Indicators in DNS Logs
Monitoring DNS logs is essential for identifying suspicious activity. Key indicators include:
- Unusual Query Lengths
- Legitimate domain names are generally short and structured.
- Abnormally long queries often signal malicious activity, such as DNS tunneling.
- High Query Frequency
- Excessive queries from a single client or to a single domain can indicate malware or botnet communication.
- Repeated Queries to Suspicious Domains
- Domains with randomized or non-standard patterns (e.g., xy12a.bcde.malicious.com) may be indicators of Command-and-Control (C2) servers.
By understanding these threats and indicators, security analysts can focus their efforts on detecting and mitigating potential risks. The next section will demonstrate how to set up the environment to begin DNS log analysis using Python.
Setting Up the Environment
To effectively analyze DNS logs, we need to prepare the necessary tools, libraries, and datasets. This section guides you through setting up a Python-based environment for DNS query analysis.
Required Tools and Libraries
We will use the following Python libraries for this analysis:
- pandas: For data manipulation and exploration.
- matplotlib and seaborn: For data visualization.
- dnslib (optional): For advanced DNS parsing and analysis.
You can install these libraries using pip:
1 | pip install pandas matplotlib seaborn dnslib |
We also recommend using a Jupyter Notebook for an interactive coding experience. If you don’t already have it installed, you can set it up with:
1 | pip install notebook |
Simulating a DNS Log Dataset
Since real DNS logs may not always be available for practice, we’ll generate a simulated dataset. This dataset will mimic real-world DNS queries and include the following fields:
- timestamp: The time when the query was made.
- query: The domain name requested.
- response_code: The DNS response status (e.g., NOERROR, NXDOMAIN).
- client_ip: The IP address of the client making the request.
Below is the Python code to generate and save the dataset:
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 | import pandas as pd import random import datetime def generate_dns_logs(num_records=1000): queries = [ "example.com", "google.com", "suspicious.exfiltration.xyz", "legitwebsite.net", "safequery.org", "this.is.a.very.long.suspicious.domain.name.that.should.get.flagged.com", "another.really.suspicious.query.that.is.too.long.for.normal.dns.usage.xyz", "maliciousdataexfiltrationtool1234567890123456789012345.com" ] response_codes = ["NOERROR", "SERVFAIL", "NXDOMAIN"] client_ips = ["192.168.1.2", "192.168.1.3", "10.0.0.5", "172.16.0.7"] timestamps = [ (datetime.datetime.now() - datetime.timedelta(minutes=i)).strftime("%Y-%m-%d %H:%M:%S") for i in range(num_records) ] data = { "timestamp": random.choices(timestamps, k=num_records), "query": random.choices(queries, k=num_records), "response_code": random.choices(response_codes, k=num_records), "client_ip": random.choices(client_ips, k=num_records) } return pd.DataFrame(data) # Create the dataset dns_logs = generate_dns_logs(1000) # Save to a CSV for reproducibility dns_logs.to_csv('dns_logs.csv', index=False) # Preview the dataset dns_logs.head() |
This script creates a .csv file (dns_logs.csv) containing simulated DNS log data, which will be used throughout the analysis.
Verifying the Environment Setup
Once the dataset is ready, load it into a Python environment to verify everything is functioning correctly:
1 2 3 4 5 6 7 8 | # Load the DNS logs from the CSV dns_logs = pd.read_csv('dns_logs.csv') # Display basic information about the dataset print(dns_logs.info()) # Preview the first few rows dns_logs.head() |
The output should display the structure of the dataset, including its columns (timestamp, query, response_code, client_ip) and a few sample rows as shown below.
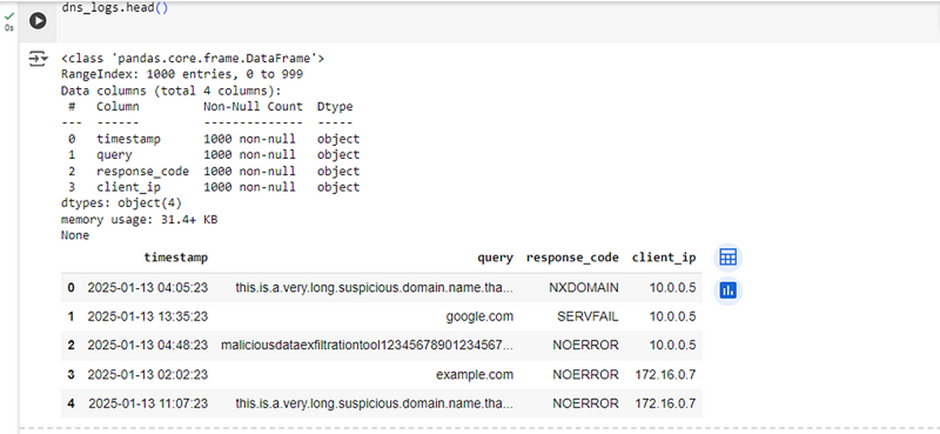
Analyzing Query Lengths
Suspicious queries often have unusually long domain names. We calculate query lengths and flag those exceeding a specified threshold:
1 2 3 4 5 6 7 8 9 | # Calculate query lengths dns_logs['query_length'] = dns_logs['query'].apply(len) # Flag queries with length > 50 as suspicious dns_logs['is_suspicious'] = dns_logs['query_length'] > 50 # Display suspicious queries suspicious_queries = dns_logs[dns_logs['is_suspicious']] suspicious_queries.head() |
Query Frequency Analysis
Analyzing query frequency helps identify patterns, such as excessive queries from a single client:
1 2 3 4 5 6 7 | # Analyze query frequency by domain query_counts = dns_logs['query'].value_counts() print(query_counts.head()) # Analyze query frequency by client IP ip_counts = dns_logs['client_ip'].value_counts() print(ip_counts.head()) |
Visualizing the Data
Distribution of Query Lengths
Visualize the distribution of query lengths using Matplotlib and Seaborn:
1 2 3 4 5 6 7 | # Visualize query lengths plt.figure(figsize=(10, 6)) sns.histplot(dns_logs['query_length'], kde=True, bins=30) plt.title('Distribution of DNS Query Lengths') plt.xlabel('Query Length') plt.ylabel('Frequency') plt.show() |
The data is displayed as shown below
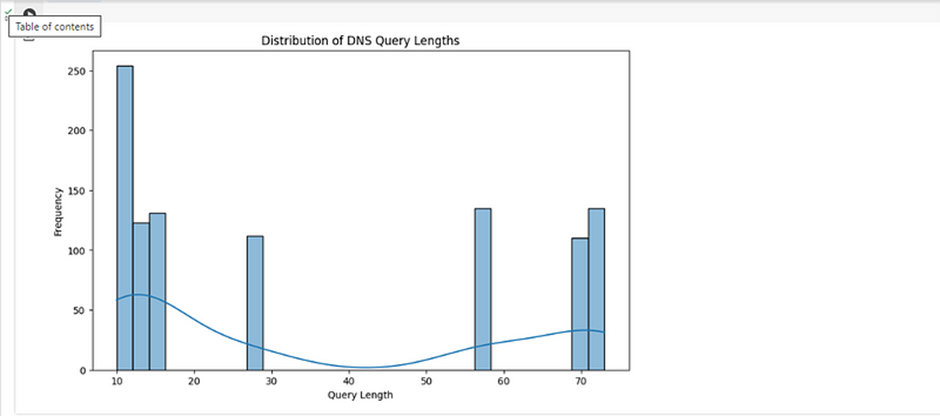
Suspicious Queries Over Time
Create a scatter plot to analyze the pattern of suspicious queries over time:
1 2 3 4 5 6 7 8 | # Scatter plot of query lengths over time plt.figure(figsize=(12, 8)) sns.scatterplot(data=dns_logs, x='timestamp', y='query_length', hue='is_suspicious', palette="viridis") plt.title('DNS Query Lengths Over Time') plt.xlabel('Timestamp') plt.ylabel('Query Length') plt.xticks(rotation=45) plt.show() |
The output shows something like this indicating the pattern of suspicious queries over time:
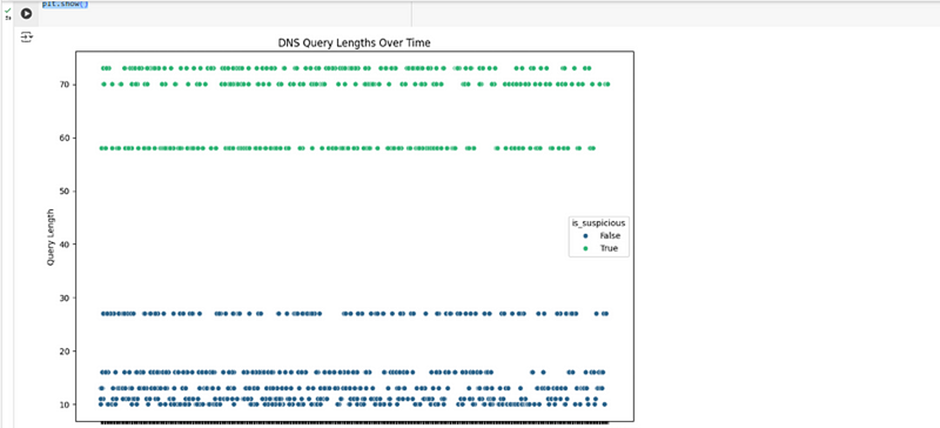
Results Summary
Summarize key findings from the analysis:
1 2 3 4 5 | # Summary statistics total_queries = len(dns_logs) total_suspicious = len(suspicious_queries) print(f"Total queries analyzed: {total_queries}") print(f"Total suspicious queries flagged: {total_suspicious}") |
Key takeaways from this analysis include:
- Total number of queries analyzed.
- Total suspicious queries flagged based on length.
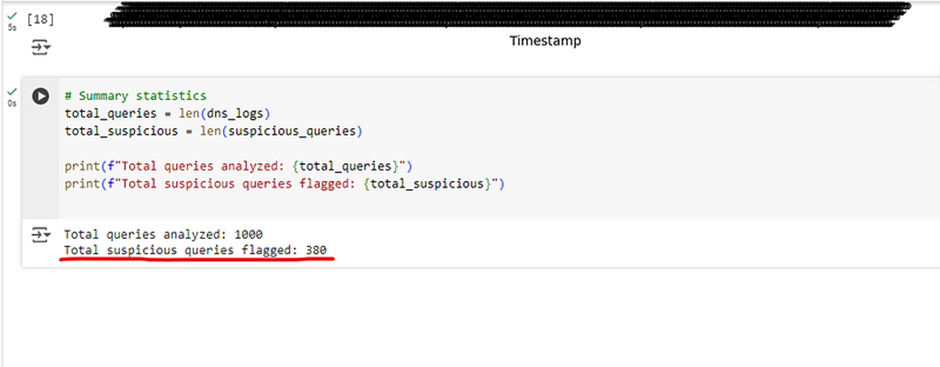
Results and Insights
Summary of Findings
We analyzed 1,000 DNS queries and flagged 380 as suspicious based on query length, with those exceeding 50 characters identified as potential threats. These flagged queries warrant further investigation as they could indicate malicious activity, such as DNS tunneling. Additionally, analysis of query frequency by domain and client IP highlighted patterns that suggest abnormal behavior.
Discussion of Limitations
The primary limitation is the potential for false positives, as legitimate long domain names can also trigger flags. Moreover, this analysis doesn’t include deeper contextual checks, such as packet inspection, which would enhance the accuracy of threat detection.
Actionable Insights
While the analysis provides useful indicators, combining DNS query data with other network logs can improve threat detection. Integrating this analysis into broader security monitoring systems will lead to more comprehensive protection.
Conclusion
DNS query analysis plays a key role in identifying security threats like DNS tunneling. With Python, we can easily flag suspicious activity and uncover potential vulnerabilities. This approach provides a solid foundation for further enhancements, such as real-time data integration and advanced detection methods, making it a powerful tool for network security.